Show:
Functional vs Object-Oriented Programming: 8 Key Differences
Functional vs object-oriented programming — two prominent programming methods, each offering a unique set of strengths and principles. Choosing which one to use will depend on your software project’s requirements. Today, we’ll explore their key differences to give you a clear picture of how they approach various aspects of software development.
We’ll discuss each essential element’s core concept, compositions, and potential pitfalls to watch out for. We also discuss some examples to learn how you can use it to achieve your goals.
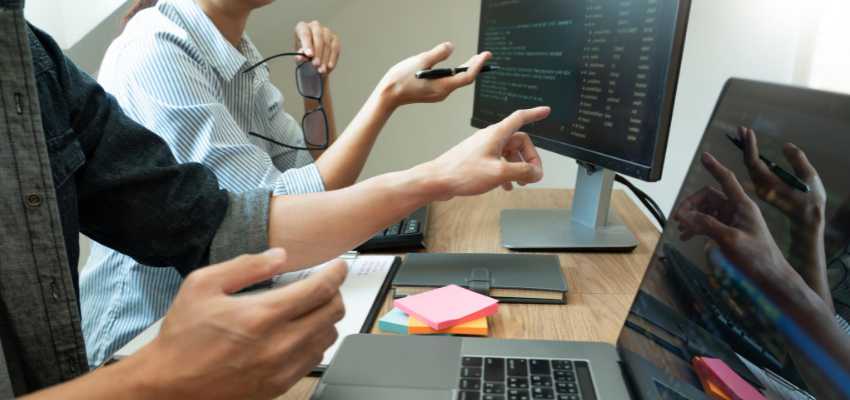
Learn about functional and object-oriented programming if you want to kickstart your programming career or advance it. This article aims to help you determine which method can provide more effective and efficient solutions for your problem. Let’s begin.
8 Useful Differences Between Functional Programming & Object-Oriented Programming
Exploring functional and object-oriented programming differences is an excellent way to improve your software quality and make strategic decisions. We also include best practices to help you enhance code quality and make it easier to maintain.
1. Composition
Functional programming (FP) focuses on using a mathematical approach to solve problems. As the name describes, it uses functions as building blocks to input data, process them, and produce results. The data you can input are numbers, text, lists, and other functions.
Most developers use FP for computation and data validation/transformation. But it can also perform any custom operations specific to your application. These operations can include anything you can express in code, from database queries to complex simulations.
If FP focuses on functions and logic, object-oriented programming (OOP) relies on objects and classes. Objects are the building blocks (basic unit) of OOP, representing a real-world entity.
On the other hand, a class defines the object’s structure, behavior, and function. It’s a simple, reusable code blueprint containing attributes (data) and functions (methods) to work. Here’s a simple example for a car class to see how it works.
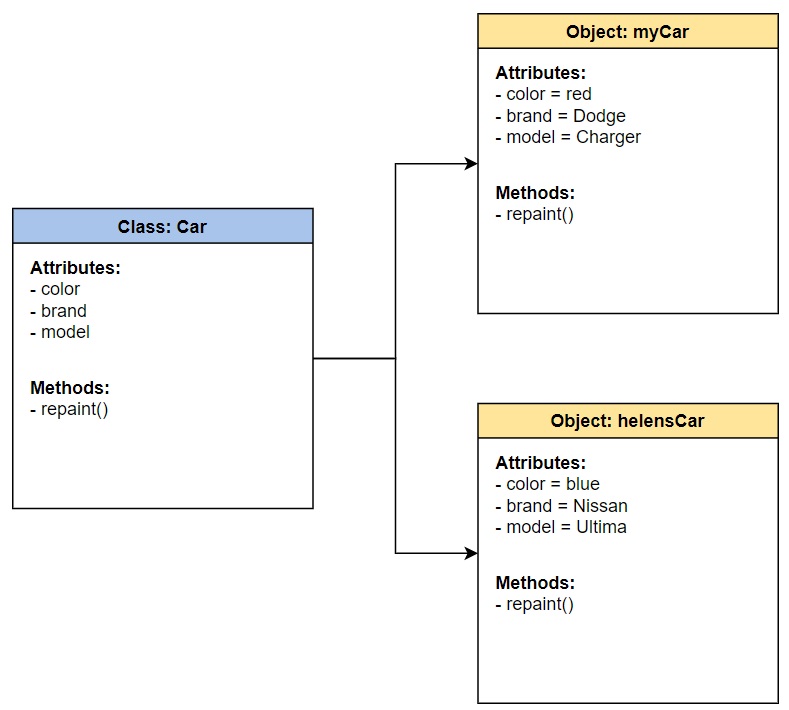
2. Core Concepts
Learning how FP and OOP build their structure helps you understand how each programming paradigm organizes and manages your code. On the other hand, core concepts will explain how they process and execute your code to achieve your desired results/goals.
2.1 Functional Programming Core Concepts
FP treats functions as first-class citizens. This enables higher-order functions and functional composition to build complex behavior from simpler, reusable components. FP’s functionality revolves around the following key concepts:
2.1.1 Higher Order Functions (HOF)
HOF is the FP concept that makes your codes adaptable and reusable. It follows 1 of 2 conditions: take one or more functions as arguments or return a function as its results. These conditions let you build small functions to build a more complex behavior. Once you create a function, you can use it in multiple contexts to fit in different parts of your codebase.
HOF also eliminates the need for hardcoding. Let’s say you’re developing a yard management system. You want to efficiently manage the allocation of parking spots for incoming trailers based on various criteria (priority, type of cargo, etc.). Typically, you need to hardcode each allocation criteria. Through HOF, you can create one function with a list of instructions on how you want to process per allocation criteria (for illustration only).
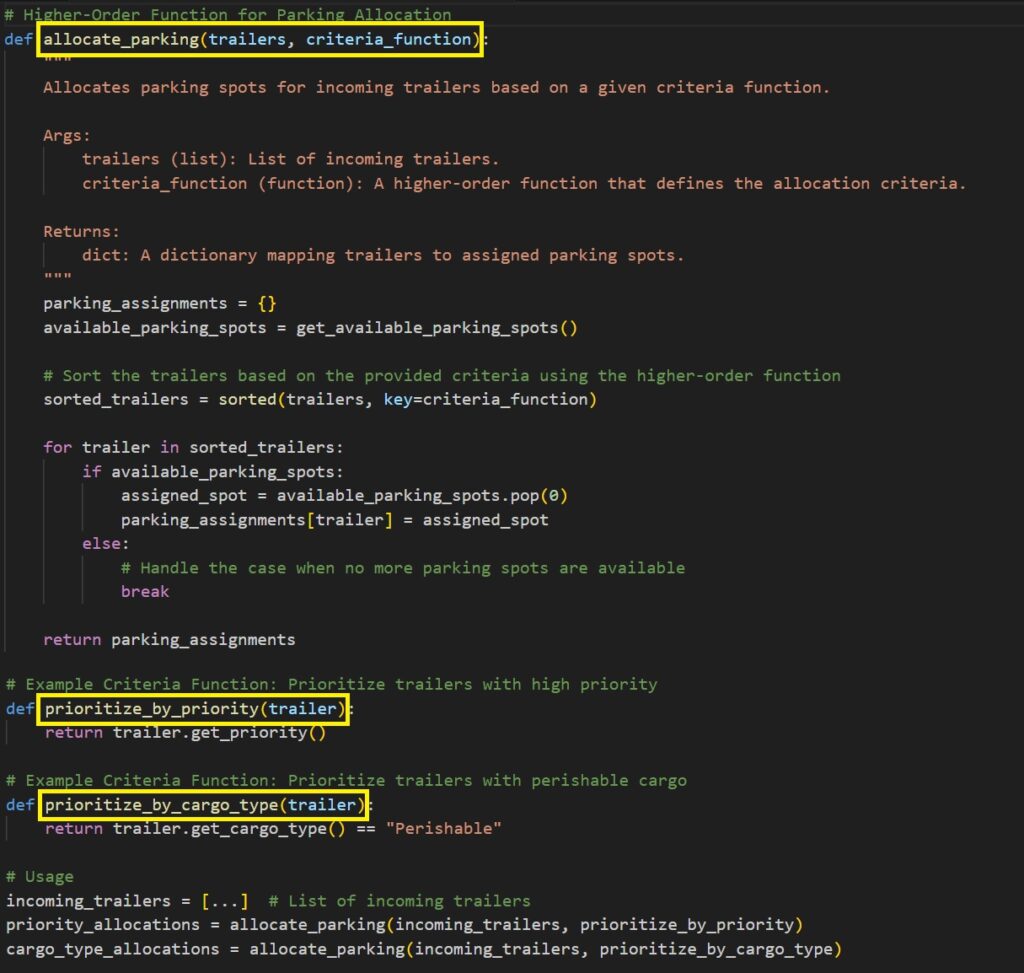
We use allocate_parking as the HOF and it consists of a list of incoming trailers and a criteria function as arguments. We also use key parameter of the sorted function to sort trailers. The prioritize_by_priority and prioritize_by_cargo_type are the sample criteria functions we create to show how you can create different allocation strategies. In short, you can use one parking allocation strategy without modifying the core allocation logic.
2.1.2 Pure Functions
Pure functions are the FP concept that minimizes unexpected behavior. It will only perform actions within its primary purpose, reducing debugging efforts. With their simplicity, you can test them without complex setups or interactions with external systems.
2.1.3 Recursion
Recursion is the FP technique that enables a function to call itself (directly or indirectly) to solve a problem. It’s a safe choice if your application performs repetitive tasks.
Every recursive function comes with one or more base cases. The base case will be the one to stop the recursive function and provide a result. The Fibonacci sequence is a classic example of recursion. The base cases it uses are F(0) = 0 and F(1) = 1. For other values of n, F(n) is computed as F(n) = F(n-1) + F(n-2).
2.2 Object-Oriented Programming Core Concepts
OOP is a superset of imperative programming (IP). IP is also a popular programming paradigm that codes every instruction or statement required to solve a specific problem. OOP shares all of its characteristics with a few extra features. One feature they share revolves around OOP core concepts:
2.2.1 Abstraction
When you write an application program, you deal with many details and instructions. Abstraction simplifies the information and focuses on what’s important. It also hides parts of your code that aren’t relevant to a current task.
2.2.2 Encapsulation
Encapsulation combines data (variables, properties) and methods. Its best example is a class, but you can also use it as access modifiers. It controls direct access to specific internal data and methods.
2.2.3 Inheritance
Inheritance is a mechanism that lets you create a new class from an existing one. The existing one will serve as the parent class, and the new ones will be the child class. As this concept’s name implies, the child class will inherit the properties and behaviors of the parent class. But it can also have its own.
2.2.4 Polymorphism
Polymorphism adds flexibility and adaptability to your codes. It enables objects to behave differently, even if they share a common method or function name. It usually occurs when you have a hierarchy of classes or parent-child classes. There are 2 methods to achieve polymorphism:
- Method overriding: Runtime polymorphism enables the child class to customize or extend the behavior of the inherited method
- Method overloading: Compile-time polymorphism allows a class to have multiple methods with the same type but a different number of parameters or vice versa
3. Data & State Management
Data and state management refers to the processes that handle, manage, and control data within an application. FP and OOP take different approaches to ensure data consistency and integrity.
The question is, which of FP and OOP handles data and state management better? The answer depends on different factors, but if you’re looking for predictability and control, here’s a comparison.
3.1 Functional Programming (FP) Wins Predictability
FP is more predictable than OOP because it uses immutable data. This means nobody can change them once you create them, ensuring the original data remains untouched. If you want to modify the data, create a new copy. You can also easily trace how data transforms from one state to another.
Additionally, FP comes with a pure function concept. So, it ensures it will always produce the same output for the same input values with no side effects. OOP is the complete opposite of FP; it relies on mutable objects. You can change the object’s internal state throughout its lifetime.
3.2 Object-Oriented Programming (OOP) Wins Control
Through OOP’s encapsulation, it provides impeccable control over data and behavior. First, it combines data and methods into a single mutable object to ensure the validity and consistency of the object’s internal state.
You can then add access modifiers — private, protected, or public. It will help specify which parts of an object’s data development team can access and modify.
You can also enforce invariants, sets of rules or conditions that must always hold true. They define the object state’s validity and consistency. You can also use these methods to run checks and error prevention.
4. Functions vs Methods
The choice between functions and methods depends on how much abstraction you need for your application. The functional programming paradigm is easier to reason about, test, and maintain. So, they are more suitable for scenarios that process data through a series of computations or transformations, such as:
- Data processing pipelines (filtering, mapping, and reducing a collection of data)
- Mathematical operations, algorithms, or computations (no state maintenance required)
- Utility functions that perform generic, reusable tasks without relying on specific objects
Object-oriented programming approaches are much more appropriate for modeling complex behaviors. Particularly to those behaviors that require state maintenance and manipulation. Here are the best applications to use these methods:
- Simulation and gaming applications
- Building user interfaces (UI) or web applications
- Objects that represent database connections, records, or tables
- Objects that represent mathematical functions, models, or algorithms
- Business applications that require to enforce business logic and rules through methods
5. Error Handling
Functional vs object-oriented programming error handling — it’s a tie. At first glance, FP looks more impressive than OOP because you can write bug-free code. It also avoids using exceptions because it promotes unpredictability over control flow. Instead, it uses FP languages to create data structures like either, maybe, or result to represent all computations that may fail.
You can use high-order functions, too. Map, filter, and reduce are a few HOFs you can build to process data. When errors arise, these HOFs can seamlessly handle them and give you a special value representing the error.
On the other hand, OOP languages (Java, C++, and Python) can handle errors through exceptions. Since this technique has unpredictable control flow, you can use Java’s try-catch blocks. The try block will define where an error might occur, and the catch block will handle the error. You have to end the code with finally statements for execution. Here’s an example.
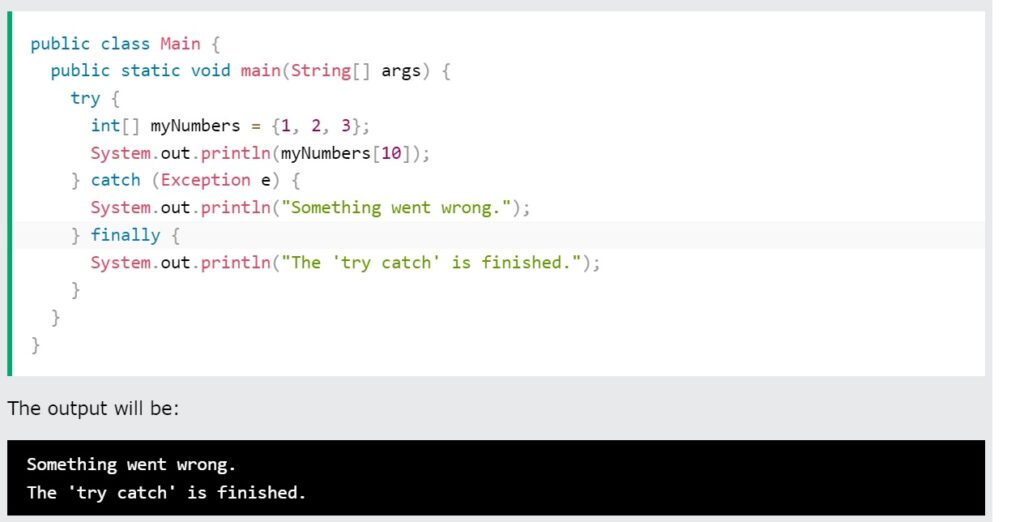
Implementing a propagation control is also an excellent solution. It gives you 2 options for handling an exception:
- At a particular level in the call stack
- Propagate it to a higher-level catch block
Regardless of your choice, both will give you control over where and how you want to handle errors. OOP is generally easy to debug because of its readable and structured code. You can spot and fix errors as you maintain the program.
6. Parallelism & Concurrency
Parallelism and concurrency may have different concepts, but they remain related. Parallelism lets a program execute multiple processes simultaneously for faster and more efficient computation.
Concurrency works the same, except the processes may overlap in time — during start, run, and completion. This holds true even if they run on a single processor. You can achieve concurrency through:
- Multitasking
- Multithreading
- Multiprocessing
- Asynchronous programming
FP can write efficient parallel and concurrent programming. Its immutability helps concurrent/parallel scenarios work on immutable data without locks or synchronization. FP languages also provide higher-order functions (e.g. map and reduce) to apply in lists or collections.
Compared to FP, OOP is neither concurrent nor parallel. That’s why they provide plenty of tools and concepts so you can implement concurrent and parallel programming efficiently.
Concurrent Programming
- Emphasize message-passing concurrency
- Define custom concurrency control mechanisms
- Concurrency libraries (create threads, manage shared resources, etc.)
- Concurrency patterns and techniques (locks or other synchronization mechanisms)
Parallel Programming
- Implement parallel algorithms
- Model parallel tasks as objects or classes
- Parallel design patterns (command, strategy, or factory)
- Parallel libraries (threading, collections, computing utilities, etc.)
7. Testing
Object-oriented and functional programming testing ensures every element and function works perfectly based on your specifications and requirements. Each modern language has its own set of testing methods. But here, I’ll show you how testing typically works in OOP & FP.
7.1 Testing Functional Programs
Testing functional programs are typically based on purity, immutability, and deterministic functions. You can start with the simplest method, unit testing — write a test for the smallest unit of your functional code.
Let’s say you want to monetize a software you created with an app membership plan. You’ve developed a functional program to automate membership management and streamline your content contribution. Using Python, here’s a simple unit test to evaluate the membership management module (for illustration only).
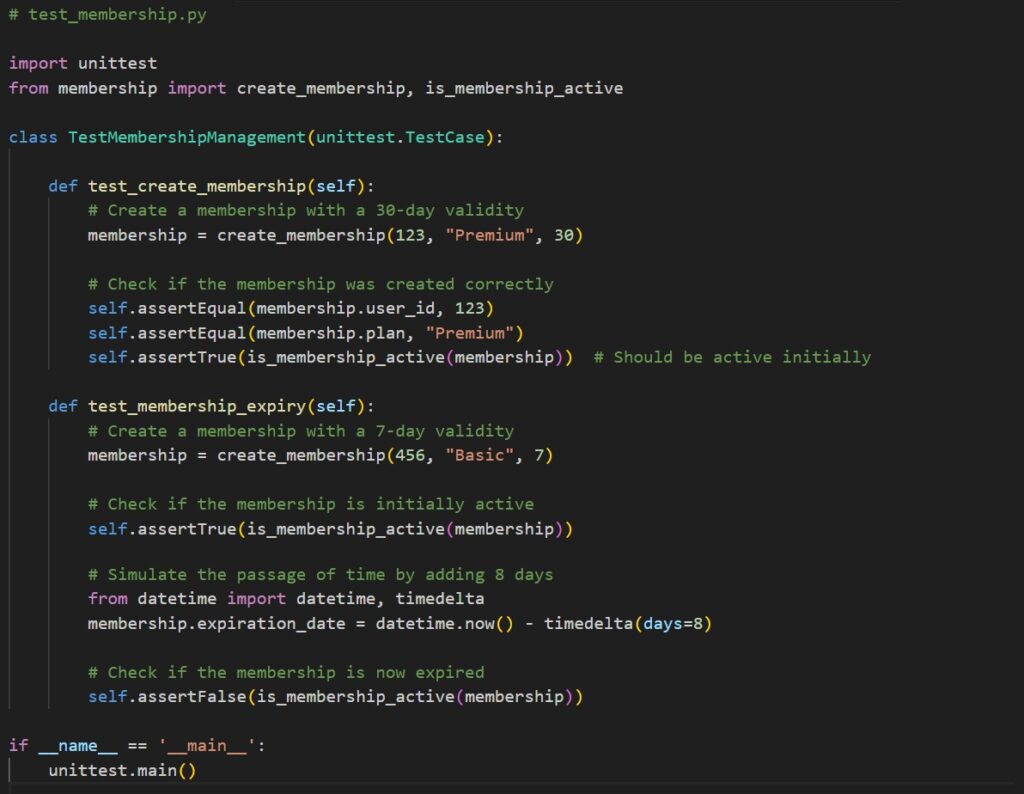
In the example, we include two test methods: test_create_membership and test_membership_expiry. The first method tests the creation process of the membership, and the other checks if a membership becomes inactive after its expiration. Here are different elements you can find:
- Write assertEqual to verify if the created memberships include the expected attributes
- Add assertTrue/assertFalse to check if memberships are active or expired as expected
- Use the import functions from the membership module so you can use them to create memberships and check their status in the test cases
Run the test_membership.py script to see if the membership management module behaves as expected. Other tests you can run for functional programs are:
- Test isolation
- Property-based testing
- Testing libraries and frameworks like QuickCheck (for Haskell) and Scalacheck (for Scala)
7.2 Testing Object-Oriented Programs
Conventional testing methods also apply to OOP because certain OOP features (abstract classes and inheritance) introduce challenges. These challenges require additional testing strategies. These strategies include:
- Fault-based testing: Identify possible faults in code or design
- Class testing based on method testing: Testing each method, relations among class methods, and inherited relation between class and subclass
- Scenario-based testing: Capture the user interactions within the application (user documentation, requirements, and reviews) and simulate them in a real-world context
8. Ease Of Use
Since OOP models real-world entities and relationships, it helps new developers to relate programming concepts to their everyday experiences. This makes it easier for them to conceptualize and design new software applications.
Scalability is another benefit of OOP. New developers can start building small, simple programs. They can gradually upgrade it to a more complex, larger program as it grows. Here are other OOP benefits that make it easier to use:
- Follow the imperative programming model
- Build application program using standard working modules
- Create error handling code separately from the normal flow
- Implement flow control using loops and conditional statements
- Strengthen security using data hiding and abstraction mechanism
FP is more challenging because of its mathematical style, particularly for developers who mostly use an imperative programming approach. But there’s nothing to worry about because its ease of use doesn’t rely on its concept. It depends on what functional programming language you choose.
Haskell is a popular programming language for FP. It’s a general-purpose programming language, but its core concept is developed purely for FP language and does well for concurrent programming. It’s also handy for type inference and lazy evaluation.
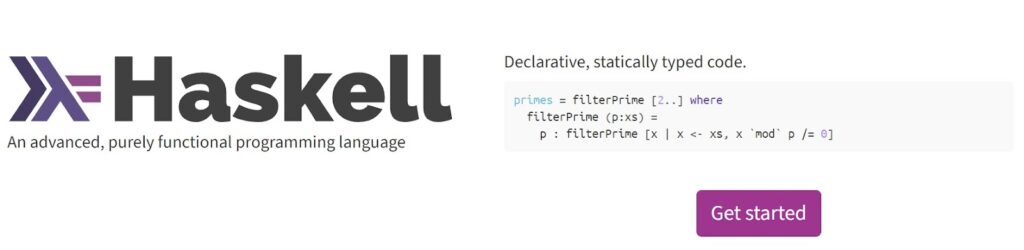
You can use Clojure, a functional programming combined with the simplicity and expressiveness of the Lisp dialect. It runs on the Java Virtual Machine (JVM) with simple integration. It’s the ideal choice for transitioning to FP. Other options you have are multi-paradigm languages, like JavaScript and Python. Overall, all FP languages can write programs using:
- Functions
- Function calls
- Function calls with recursion
Conclusion
Discussing the difference between functional vs object-oriented programming is not to determine a winner. It’s about understanding each programming paradigm’s strengths and knowing when to use them to build better software programs.
Functional and object-oriented programming have different programming styles, but they share a common goal — writing bug-free code that’s easy to build and maintain. Functional programming focuses on predictability and security. Its declarative style of coding also makes it easy for developers to describe what they want to achieve instead of specifying step-by-step instructions on how to achieve it.
Object-oriented programming addresses this goal through encapsulation and abstraction, which promotes reusability and modularity. Whichever option you choose, make sure it aligns with your project’s specific requirements and goals.
Are you ready to turn your dream project to life? AxiomQ can make it happen. Our team has the creative spark and technical expertise to sketch new software from scratch or fine-tune existing applications. Visit our website, and let’s collaborate.
About the author:
Burkhard Berger is the founder of Novum™. He helps innovative B2B companies implement revenue-driven SEO strategies to scale their organic traffic to 1,000,000+ visitors per month. Curious about what your true traffic potential is?